What is Diva Staking?
Think of Diva Staking as your personal factory for creating staking platforms. Just like Shopify lets anyone launch their own online store, Diva Staking lets you launch your own liquid staking protocol on Solana.
In Simple Terms:
- You get all the tools to run your own staking service
- Create your own staking token that users can freely trade
- Set your own rules, fees, and build your community
Traditional Staking vs Liquid Staking Protocol
Traditional Staking
- Tokens are locked
- Limited validator choice
- No additional yield opportunities
Your Liquid Staking Protocol
- Instant liquidity with tradeable tokens
- Automated validator management
- Additional DeFi opportunities
Everything You Need to Launch
Our platform provides all the tools and features needed to create and manage your own liquid staking protocol.
Customizable Protocol
Design your staking protocol with custom parameters, fees, and governance structure.
Automated Management
Smart contracts handle validator selection, delegation, and reward distribution automatically.
Liquid Staking Tokens
Issue tradeable tokens that represent staked assets, enabling DeFi integration.
Security First
Built-in security measures protect against validator misbehavior and smart contract risks.
Analytics Dashboard
Monitor protocol performance, validator metrics, and user activity in real-time.
Community Tools
Built-in features for governance, community engagement, and protocol growth.
Core Features Deep Dive
Explore the powerful features that make Diva Staking the most advanced liquid staking platform.
Distributed Validator Technology (DVT)
Our advanced validator management system ensures maximum security and performance through distributed validation.
Technical Implementation
struct DistributedValidatorConfig { threshold: u8, // Consensus threshold total_validators: u8, // Total validator count min_stake: u64, // Min stake per validator fault_tolerance: u8, // Fault tolerance level }
- • BLS signature aggregation for efficient consensus
- • Threshold Signature Scheme (TSS) implementation
- • Automated stake rebalancing algorithm
- • Real-time performance monitoring and metrics
Token Economics & Fee Structure
Customizable tokenomics and fee parameters to align with your business model and community needs.
Technical Implementation
interface TokenConfig { mint_authority: PublicKey fee_structure: { deposit_fee: BasisPoints withdrawal_fee: BasisPoints performance_fee: BasisPoints } rebase_frequency: number treasury_account: PublicKey }
- • SPL Token standard implementation
- • Automated rebase mechanism
- • MEV-resistant fee collection
- • Real-time APY calculations
Security Systems
Enterprise-grade security with multiple layers of protection and monitoring.
Technical Implementation
interface SecuritySystem { monitoring: { anomaly_detection: boolean performance_tracking: boolean threat_detection: boolean } protection: { mev_protection: boolean slashing_protection: boolean ddos_protection: boolean } }
- • Circuit breaker implementation
- • Rate limiting and access control
- • Automated security auditing
- • Incident response automation
Protocol Management Tools
Comprehensive dashboard and tools for managing your protocol with ease.
Technical Implementation
interface ManagementTools { dashboard: { analytics: boolean monitoring: boolean alerts: boolean } controls: { parameter_adjustment: boolean emergency_shutdown: boolean validator_management: boolean } reporting: ReportingSystem }
- • GraphQL API for data access
- • WebSocket real-time updates
- • Automated health checks
- • Custom alert configurations
Technical Architecture
A deep dive into the technical components that power our liquid staking infrastructure.
Protocol Layer
- • Stake Pool Management
- • Fee Management
- • Governance System
Validator Layer
- • DVT System
- • Performance Monitoring
- • Slashing Protection
Token Layer
- • LST Management
- • Price Oracle
- • Reward System
Implementation Examples
pub struct StakePool { pub version: u8, pub manager: Pubkey, pub stake_deposit_authority: Pubkey, pub stake_withdraw_bump_seed: u8, pub validator_list: Pubkey, pub reserve_stake: Pubkey, pub pool_mint: Pubkey, pub manager_fee_account: Pubkey, pub token_program_id: Pubkey, pub total_stake: u64, pub pool_token_supply: u64, pub last_update_epoch: u64, pub lockup: Lockup, pub fee: Fee, }
async function mintLiquidToken( pool: StakePool, amount: number, recipient: PublicKey ): Promise<TransactionSignature> { const mint_ix = createMintToInstruction( pool.pool_mint, recipient, pool.manager, amount ); return await sendAndConfirmTransaction( connection, new Transaction().add(mint_ix), [manager] ); }
Performance Metrics
Transaction Speed
Latency
Uptime
Success Rate
Security Framework
Enterprise-grade security infrastructure protecting your protocol at every layer.
Multi-layer Security
Comprehensive security at every level of the protocol stack.
Protocol Layer
- Smart contract security & audits
- Formal verification
Network Layer
- DDoS protection
- Secure RPC endpoints
Application Layer
- Access control systems
- Rate limiting & circuit breakers
Security Implementation
{ "security_layers": { "protocol": { "audits": true, "formal_verification": true, "upgrade_timelock": 48 // Hours }, "network": { "ddos_protection": true, "rpc_security": { "rate_limit": 1000, // Requests/min "whitelist": true } }, "application": { "circuit_breaker": { "tvl_change": 10, // Percent "tx_volume": 1000000 // USD } } } }
Risk Management Systems
Proactive risk mitigation and automated safety measures.
- Automated Circuit Breakers
Instant protocol pause on suspicious activity detection
- Stake Concentration Limits
Enforced diversification across validators
- Insurance Coverage
Optional coverage for staked assets
Risk Management Config
{ "risk_management": { "circuit_breakers": { "tvl_change": { "threshold": 10, // Percent "timeframe": 3600 // Seconds }, "transaction_volume": { "threshold": 1000000, // USD "timeframe": 300 // Seconds } }, "stake_limits": { "max_per_validator": 100000, // SOL "min_validators": 5, "max_concentration": 20 // Percent }, "insurance": { "coverage_ratio": 100, // Percent "premium": 0.1, // Percent "claim_period": 7 // Days } } }
Monitoring & Alert Systems
24/7 monitoring with real-time alerts and automated responses.
- Real-time Monitoring
Continuous tracking of protocol metrics and performance
- Automated Alerts
Instant notifications for critical events
- Incident Response
Automated mitigation and response procedures
Monitoring Configuration
{ "monitoring": { "metrics": { "performance": { "frequency": 60, // Seconds "thresholds": { "latency": 1000, // ms "error_rate": 0.1 // Percent } }, "health": { "frequency": 300, // Seconds "checks": [ "validator_status", "network_status", "tvl_changes" ] } }, "alerts": { "channels": [ "slack", "email", "pager" ], "severity_levels": { "critical": true, "warning": true, "info": true } } } }
Audit Reports & Certifications
Comprehensive security validations from industry leaders.
CertiK Audit
Smart Contract Security
Hacken Audit
Platform Security
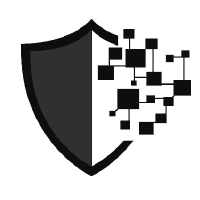
SlowMist Audit
Infrastructure Security
Security Metrics
Recent Security Updates
- Quarterly audit completed (Q1 2025)
- Security patches implemented
- Monitoring system upgraded
Protocol Governance
Decentralized governance framework ensuring transparent and community-driven protocol evolution
DAO Structure
Multi-tiered governance structure with balanced representation and clear decision-making processes.
Protocol Council
Elected representatives responsible for high-level protocol decisions and emergency responses.
Technical Committee
Expert group overseeing protocol upgrades and technical implementations.
Community Assembly
Open forum for discussion, proposal submission, and community feedback.
DAO Implementation
pub struct DAOStructure { // Governance bodies pub council: Council, pub technical_committee: TechnicalCommittee, pub community: CommunityAssembly, // Membership management pub members: Members, pub roles: Roles, // Decision thresholds pub thresholds: DecisionThresholds, } impl DAOStructure { pub fn propose_action( &self, action: GovernanceAction, proposer: Member ) -> Result<Proposal> { // Create and validate proposal } pub fn execute_decision( &self, decision: Decision ) -> Result<Execution> { // Execute approved decision } }
Voting Mechanism
Secure and transparent voting system with multiple voting strategies and delegation options.
Token-Weighted Voting
Voting power proportional to token holdings with optional time-locking multipliers.
Delegation System
Flexible delegation options allowing users to delegate voting power to trusted representatives.
Quadratic Voting
Advanced voting mechanism to prevent plutocracy and ensure fair representation.
Voting System Implementation
pub struct VotingMechanism { // Voting configurations pub voting_power: VotingPower, pub delegation: DelegationSystem, pub quadratic: QuadraticVoting, // Vote tracking pub votes: Votes, pub results: VoteResults, } impl VotingMechanism { pub fn cast_vote( &self, voter: Voter, proposal: Proposal, vote: Vote ) -> Result<VoteReceipt> { // Process and record vote } pub fn calculate_results( &self, proposal: Proposal ) -> Result<VoteOutcome> { // Calculate final vote results } }
Upgrade Process
Structured protocol upgrade process with multiple safety checks and community involvement.
Upgrade Implementation
pub struct UpgradeProcess { // Upgrade stages pub proposal: UpgradeProposal, pub review: TechnicalReview, pub testing: TestingPhase, pub deployment: Deployment, // Safety measures pub timelock: Timelock, pub emergency_brake: EmergencyBrake, } impl UpgradeProcess { pub async fn initiate_upgrade( &self, upgrade: Upgrade ) -> Result<UpgradeStatus> { // Start upgrade process } pub fn verify_upgrade( &self, upgrade: Upgrade ) -> Result<VerificationStatus> { // Verify upgrade safety } }
Community Participation
Multiple channels for community engagement and contribution to protocol development.
Discussion Forums
Active community forums for proposal discussion and feedback.
Working Groups
Specialized teams focusing on specific protocol aspects.
Bounty Program
Rewards for community contributions and improvements.
Community System Implementation
pub struct CommunitySystem { // Participation channels pub forums: DiscussionForums, pub working_groups: WorkingGroups, pub bounties: BountyProgram, // Engagement tracking pub metrics: EngagementMetrics, pub rewards: RewardSystem, } impl CommunitySystem { pub fn submit_contribution( &self, contributor: Member, contribution: Contribution ) -> Result<ContributionStatus> { // Process community contribution } pub fn distribute_rewards( &self, period: RewardPeriod ) -> Result<RewardDistribution> { // Calculate and distribute rewards } }
Launch Process
Your comprehensive guide to launching a liquid staking protocol with our infrastructure
Step-by-step Guide
Clear and structured process to launch your liquid staking protocol
Initial Setup
Configure protocol parameters and customize token economics
Validator Selection
Choose and configure your validator set with DVT implementation
Testing Phase
Comprehensive testing on testnet with simulated scenarios
Mainnet Launch
Controlled deployment with gradual stake limit increase
Launch Process Implementation
pub struct LaunchProcess { // Launch stages pub setup: InitialSetup, pub validators: ValidatorSetup, pub testing: TestingPhase, pub deployment: MainnetDeployment, // Progress tracking pub progress: LaunchProgress, pub metrics: LaunchMetrics, } impl LaunchProcess { pub async fn initialize_protocol( &self, config: ProtocolConfig ) -> Result<ProtocolInstance> { // Initialize protocol with config } pub fn track_progress( &self ) -> LaunchStatus { // Monitor launch progress } }
Requirements Checklist
Essential requirements and prerequisites for a successful launch
Technical Requirements
Infrastructure setup and technical specifications
Security Measures
Security protocols and monitoring systems
Legal Compliance
Regulatory requirements and compliance documentation
Community Readiness
Community engagement and support systems
Requirements Implementation
pub struct LaunchRequirements { // Technical requirements pub infrastructure: InfrastructureReqs, pub security: SecurityReqs, pub monitoring: MonitoringReqs, // Compliance requirements pub legal: LegalRequirements, pub regulatory: RegulatoryReqs, // Community requirements pub community: CommunityReqs, pub support: SupportSystem, } impl LaunchRequirements { pub fn verify_requirements( &self ) -> Result<RequirementStatus> { // Verify all requirements } pub fn generate_report( &self ) -> RequirementsReport { // Generate compliance report } }
Timeline Expectations
Estimated timeline for each phase of the launch process
Initial Setup
Protocol configuration and customization
Testing Phase
Comprehensive testing and validation
Security Audit
External security review and fixes
Launch
Mainnet deployment and monitoring
Timeline Implementation
pub struct LaunchTimeline { // Timeline phases pub setup_phase: Phase, pub testing_phase: Phase, pub audit_phase: Phase, pub launch_phase: Phase, // Progress tracking pub current_phase: PhaseStatus, pub estimated_completion: DateTime, } impl LaunchTimeline { pub fn update_progress( &mut self, phase: Phase, status: Status ) -> Result<TimelineUpdate> { // Update phase progress } pub fn get_estimated_completion( &self ) -> DateTime { // Calculate completion date } }
Support Process
Comprehensive support system throughout your launch journey
Technical Support
Expert assistance for technical implementation and troubleshooting
Launch Guidance
Strategic advice and best practices for successful launch
Community Support
Assistance with community building and engagement strategies
Support System Implementation
pub struct SupportSystem { // Support channels pub technical: TechnicalSupport, pub strategic: LaunchSupport, pub community: CommunitySupport, // Metrics tracking pub response_time: Metrics, pub resolution_rate: Metrics, pub satisfaction: Metrics, } impl SupportSystem { pub async fn create_ticket( &self, issue: SupportIssue ) -> Result<Ticket> { // Create support ticket } pub fn get_support_status( &self, ticket: TicketId ) -> TicketStatus { // Get ticket status } }
Developer Resources
Everything you need to build and integrate with Diva Staking Protocol
Quick Start Guide
Get up and running with Diva Staking in less than 10 minutes
npm install @diva-staking/sdk
import { DivaProtocol } from '@diva-staking/sdk'
API Documentation
Comprehensive API reference with examples and use cases
SDK Overview
Powerful tools and utilities for protocol integration
- • TypeScript/JavaScript Support
- • Solana Web3.js Integration
- • Advanced Transaction Building
- • Event Handling & Monitoring
Implementation Examples
Ready-to-use implementation patterns for different components
pub struct StakePool {
pub version: u8,
pub manager: Pubkey,
pub stake_deposit_authority: Pubkey,
pub stake_withdraw_bump_seed: u8,
pub validator_list: Pubkey,
pub reserve_stake: Pubkey,
pub pool_mint: Pubkey,
pub manager_fee_account: Pubkey,
pub token_program_id: Pubkey,
pub total_stake: u64,
pub pool_token_supply: u64,
pub last_update_epoch: u64,
pub lockup: Lockup,
pub fee: Fee,
}
async function mintLiquidToken(
pool: StakePool,
amount: number,
recipient: PublicKey
): Promise<TransactionSignature> {
const mint_ix = createMintToInstruction(
pool.pool_mint,
recipient,
pool.manager,
amount
);
return await sendAndConfirmTransaction(
connection,
new Transaction().add(mint_ix),
[manager]
);
}
Platform Comparison
See how Diva Staking compares to other staking solutions
Vs. Traditional Staking
Key advantages over traditional staking methods
Liquidity
Immediate access to staked assets through liquid tokens vs. locked staking periods
Yield Optimization
Advanced yield strategies vs. basic staking rewards
Risk Distribution
Distributed validator technology vs. single validator risk
Performance Comparison
Feature Comparison Matrix
Detailed comparison of features across different staking solutions
Features | Diva Staking | Traditional | Other LSDs | Custom Build |
---|---|---|---|---|
Liquid Tokens | ✓ | ✗ | ✓ | ✓ |
MEV Protection | ✓ | ✗ | ✗ | ✓ |
DVT | ✓ | ✗ | ✓ | ✗ |
Custom Parameters | ✓ | ✗ | ✗ | ✓ |
Enterprise Support | ✓ | ✗ | ✗ | ✓ |
Quick Deployment | ✓ | ✓ | ✓ | ✗ |
Cost Effective | ✓ | ✓ | ✓ | ✗ |
Success Stories
See how organizations are using Diva Staking to transform their liquid staking operations
Major Exchange Integration
Leading crypto exchange
"Diva Staking's infrastructure allowed us to launch our own liquid staking protocol in weeks instead of months. The security and scalability exceeded our expectations."
DAO Treasury Management
Top DeFi protocol
"The customizable parameters and governance features made it perfect for our DAO's needs. We're now generating additional yield on our treasury assets."
Future Development
Discover how we're shaping the future of liquid staking through innovation and collaboration
Roadmap
Our strategic plan for expanding protocol capabilities and ecosystem reach
Q1 2025: Layer 2 Integration
Scaling solutions for faster, cheaper transactions
// Example L2 Bridge Integration async function bridgeToL2(amount: BigNumber) { const bridge = new L2Bridge(config) return bridge.deposit({ amount, token: "stSOL", destination: L2_CONTRACT }) }
Q2 2025: Cross-Chain Infrastructure
Unified staking across multiple blockchains
// Cross-Chain Message Passing interface CrossChainMessage { source: Chain target: Chain payload: Bytes proof: MerkleProof }
Technical Documentation
Detailed specifications and implementation guides
Upcoming Features
Next-generation capabilities being developed for the protocol
Advanced Analytics Dashboard
Real-time metrics and performance insights
interface AnalyticsDashboard { metrics: { apy: number tvl: BigNumber validators: number } performance: { uptime: number latency: number } }
Multi-Token Support
Stake and manage multiple token types
// Multi-Token Staking Pool class MultiTokenPool { async stake(token: TokenType, amount: BigNumber) { const rate = await this.getExchangeRate(token) return this.mint(amount.mul(rate)) } }
Feature Documentation
Implementation guides and API references
Research & Development
Cutting-edge research initiatives pushing the boundaries of staking technology
Zero-Knowledge Proofs
Implementing privacy-preserving staking operations using zk-SNARKs
// ZK Proof Generation async function generateProof( secret: Scalar, commitment: Point ): Promise<ZKProof> { return prove(circuit, { secret, commitment, nullifier: hash(secret) }) }
Quantum Resistance
Future-proofing protocol security against quantum computing threats
// Quantum-Safe Signatures class QuantumSafeValidator { sign(message: Bytes): Signature { return this.dilithium.sign( message, this.keyPair ) } }
Research Papers
Academic publications and technical specifications
Partnership Opportunities
Collaborate with us to build the future of decentralized staking
Protocol Integrations
Seamlessly integrate liquid staking into your DeFi protocol
// Integration Example class ProtocolIntegration { async integrateStaking( protocol: Protocol, config: StakeConfig ): Promise<void> { await this.setupHooks(protocol) await this.initializePool(config) } }
Enterprise Solutions
Custom implementations for institutional needs
// Enterprise Setup interface EnterpriseConfig { compliance: ComplianceLevel reporting: ReportingConfig custody: CustodyType limits: { daily: BigNumber total: BigNumber } }
Partnership Guide
Learn how to become a partner